What is Apache Spark?
Apache Spark is an open-source distributed computing system primarily used for big data processing and analytics. It was developed to improve the speed and simplicity of big data analysis by providing an easy-to-use interface and in-built capabilities for distributed data processing. Spark can process data from various sources such as Hadoop Distributed File System (HDFS), Apache Cassandra, Apache HBase, and S3, among others.
One of Spark’s defining features is its ability to perform in-memory computations, drastically reducing the time required for iterative tasks and interactive queries. The system leverages resilient distributed datasets (RDDs) to store data in memory, minimizing the need for disk I/O and significantly speeding up analytics processes. Furthermore, Spark supports multiple programming languages including Java, Python, Scala, and R, broadening its accessibility to data engineers and data scientists.
Learn more in our detailed guide Apache Spark tutorial.
Understanding Apache Spark concepts
Resilient Distributed Datasets (RDDs)
Resilient distributed datasets (RDDs) are fundamental to Apache Spark. They are immutable and distributed collections of objects that can be processed in parallel. An RDD can be created from a local collection, or from datasets stored in external storage systems like HDFS, HBase, and S3.
Immutability of RDDs ensures fault tolerance by maintaining lineage information which tracks the sequence of operations that produced them. If a node fails, Spark uses the lineage to recompute lost data rather than replicating it across nodes. This approach not only reduces the replication overhead but also enhances data recovery speed.
Partition
Partitioning is essential for efficiently distributing data across the cluster. An RDD is divided into multiple partitions, each processed by a separate task. This method facilitates parallelism, leveraging the cluster’s computational power effectively.
Each partition resides on a different node in the cluster, and the number of partitions is governed by the cluster configuration and the dataset size. Managing partitions effectively can optimize resource utilization, reduce latency, and improve overall processing time of the data.
Transformation
Transformations in Spark are operations applied to RDDs to create a new RDD. Common transformations include map()
, filter()
, and reduceByKey()
. These operations are lazily evaluated, meaning they don’t execute immediately but are instead recorded in a lineage graph.
Lazy evaluation ensures that Spark optimizes the entire execution plan rather than executing operations one by one. When an action is called, Spark utilizes this lineage graph to build an efficient query execution plan, significantly reducing computational overhead.
Action
Actions trigger the execution of transformations to return results to the driver program or to write data to an external storage system. Examples of actions include collect()
, count()
, and saveAsTextFile()
. Unlike transformations, actions are executed immediately.
Actions play a critical role in materializing the computations applied on RDDs. They begin the data processing workflow and aggregate the results after all transformations are applied. Spark optimizes the execution by pipelining transformations and converting them into a series of execution tasks submitted to the cluster.
Directed Acyclic Graphs (DAG)
Directed acyclic graphs (DAGs) optimize the execution plan in Spark. When a transformation is performed, Spark constructs a DAG of stages that represent a sequence of computation steps derived from RDD operations. This graph structure ensures there are no cycles, which otherwise could lead to infinite loops.
DAGs contribute to fault tolerance and efficient job scheduling. By splitting tasks into stages, Spark can recover from failures by rerunning only the affected stages rather than the entire job, resulting in improved reliability and fault tolerance.
Datasets and DataFrames
Datasets and DataFrames are higher-level APIs that offer expressive data processing capabilities over RDDs. They provide a structured way to work with semi-structured and structured data, integrating schema information into the processing tasks.
Datasets are strongly-typed using JVM objects and provide compile-time type safety. DataFrames, on the other hand, are untyped, and their schema is represented by a collection of rows and columns, similar to a relational database. This abstraction simplifies complex data manipulations and optimizes execution through Catalyst optimizers.
Tasks
In Spark, tasks are units of work sent to worker nodes for execution. Each task operates on a partition of data, leveraging the distributed nature of the system to parallelize computations. Tasks are fundamental in the execution of transformations and actions defined on RDDs.
Detailed task tracking ensures fault tolerance and load balancing. If a task fails or straggles, Spark can reassign it to another node for re-execution. Effective task scheduling and execution management can significantly boost the speed of data processing workflows.
Learn more about Data architecture principles
Spark architecture and components
The Spark Driver
The Spark driver is the heart of a Spark application. It maintains the application’s lifecycle, scheduling tasks and managing job execution across the cluster. The driver converts user-defined transformations and actions into a logical execution plan using DAGs.
The driver coordinates the execution of the DAG, monitors job status, and collects metrics. It communicates with the cluster manager to allocate resources, dispatch tasks to executors, and retrieve results. A robust driver is essential for maintaining scalability and fault tolerance in Spark applications.
The Spark Executors
Spark executors are responsible for executing tasks assigned by the driver. Each executor runs on a worker node and maintains a subset of data partitions in memory. Executors perform computational tasks on these partitions, caching intermediate results to speed up iterative operations.
Executors also handle data exchange between nodes, facilitating distributed data processing. They regularly report the status of their tasks to the driver, helping it track progress and handle faults or stragglers. Efficient executor management optimizes resource utilization and speeds up job execution.
The Cluster Manager
The cluster manager coordinates resource allocation for Spark applications. It interacts with the driver to manage node allocation, task scheduling, and resource distribution across the cluster. Popular cluster managers include Apache YARN, Mesos, and Kubernetes.
The cluster manager is pivotal for maintaining optimal resource usage and ensuring workloads are evenly distributed. It dynamically adjusts resource allocation based on current demand and job requirements, providing a flexible and scalable environment for Spark applications.
Worker Nodes
Worker nodes are the physical machines in a Spark cluster that execute the tasks assigned by the driver. Each worker node runs one or more executors to perform data processing and storage. They manage task execution, data caching, and inter-node communication.
Efficient utilization of worker nodes is critical for maximizing the throughput and performance of Spark jobs. Ensuring a balanced distribution of tasks and optimal resource allocation helps maintain high performance and fault tolerance in a Spark cluster.
sparkContext
sparkContext is the entry point for a Spark application. It allows users to interact with the Spark cluster, creating RDDs and enabling data manipulations. It coordinates the execution of operations and communicates with the cluster manager and nodes.
By managing application configuration and resource allocation, sparkContext plays a central role in the overall execution framework. It initializes essential services and monitors the application’s lifecycle, ensuring smooth operation and efficient resource usage.
Spark Core
Spark Core is the foundational engine underpinning all Spark functionalities. It provides essential services like task scheduling, memory management, fault recovery, and job execution. Spark Core’s architecture ensures efficient handling of big data workloads.
The core library includes APIs for manipulating RDDs, facilitating transformations, and executing actions. It integrates with higher-level Spark components like SQL, Streaming, and MLlib, offering a cohesive platform for various big data applications. Effective management of Spark Core is crucial for optimizing Spark’s performance and scalability.
Spark SQL
Spark SQL is the module for structured data processing. It allows querying data via SQL and integrating the relational processing with Spark’s procedural API. Spark SQL provides a SQL-like interface to work with structured and semi-structured data.
By leveraging the Catalyst optimizer, Spark SQL enhances query performance and enables more efficient data processing. Users can blend SQL queries with RDD transformations, enabling powerful data analytics and manipulation capabilities within a single framework.
Spark Streaming
Spark Streaming extends the core Spark API to support scalable, high-throughput, fault-tolerant stream processing of live data streams. Data can be ingested from various sources like Kafka, Flume, and HDFS, and processed using complex algorithms expressed with high-level functions like map, reduce, join, and window.
Spark Streaming processes data in mini-batches, providing the benefits of batch processing and real-time processing. It achieves fault tolerance through Spark’s RDD abstraction and can recover from node failures, making it reliable for critical applications. Integrating with other Spark components, Spark Streaming enables powerful and unified data processing capabilities.
MLlib
MLlib is Spark’s scalable machine learning library. It provides a rich set of tools including algorithms for classification, regression, clustering, and collaborative filtering. Additionally, it offers utilities for feature extraction, transformation, dimensionality reduction, and selection.
By leveraging Spark’s distributed computing capabilities, MLlib enables scalable and efficient machine learning on large datasets. It integrates with Spark’s core and other libraries, allowing users to build complex machine learning pipelines combining different data processing and analytics tools.
GraphX
GraphX is Spark’s API for graph processing and analysis. It allows users to create and manipulate graphs, providing a range of operators for graph computation such as subgraph, joinVertices, and aggregateMessages. GraphX also includes a library of common graph algorithms like PageRank, Connected Components, and Triangle Counting.
GraphX combines the advantages of both graph-parallel and data-parallel systems. By representing graphs as RDDs, it leverages Spark’s execution engine for fault tolerance and scalability. This integration enables users to perform graph computation along with data processing tasks within a unified framework.
Spark APIs
Spark APIs provide a suite of interfaces for interacting with Spark. The primary languages supported are Scala, Java, Python, and R. Each API is designed to provide the full capabilities of Spark, allowing users to write applications in their preferred language.
The APIs support a wide range of operations, including data manipulation, query execution, and machine learning. By offering consistent functionality across different languages, Spark APIs enable developers to leverage Spark’s power without being limited by programming language constraints. This flexibility helps in building versatile big data applications that can address diverse analytical needs.
Learn more in our detailed guide to Apache Spark tuning.
Tips from the expert
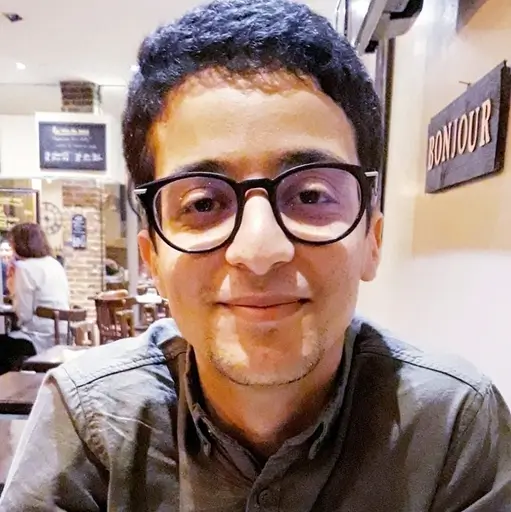
Ahmed Khaldi
Solution Architect
Ahmed Khaldi is Solutions Architect with extensive experience in Apache SPARK and a proven track record in optimizing cloud infrastructure for various teams including DevOps, FinOps, SecOps, and ITOps.
In my experience, here are tips that can help you make the most of Apache Spark architecture:
- Leverage advanced memory tuning: Beyond basic caching, experiment with memory fractions (
spark.memory.fraction, spark.memory.storageFraction
) to optimize the balance between execution and storage memory. Fine-tuning these parameters can prevent excessive garbage collection and improve job stability. - Optimize data serialization: Use Kryo serialization instead of Java serialization (
spark.serializer
). Kryo is more efficient and can significantly reduce the serialization overhead, especially with large and complex data structures. - Strategic use of coalesce and repartition: While repartition increases parallelism, it can be costly due to shuffling. Use
coalesce()
to reduce the number of partitions efficiently without a full shuffle, particularly after filtering operations that reduce dataset size. - Implement efficient join strategies: For large-scale joins, consider using broadcast joins (
broadcast
function) to distribute smaller datasets across all nodes, thus avoiding shuffling large datasets. Optimize join performance by ensuring the join keys are well-distributed. - Utilize custom partitioners: For operations that involve repeated shuffling, such as aggregations and joins, creating custom partitioners can ensure data is distributed in a way that minimizes shuffling and balances workload across the cluster.
Best practices for designing Apache Spark applications
Optimize Data Partitioning
Effective data partitioning is crucial for maximizing the performance and scalability of your Spark application. When data is unevenly distributed across partitions, it can lead to skewed workloads where some nodes are overburdened while others are underutilized, causing bottlenecks and inefficiencies.
To avoid these issues, carefully design your partitioning strategy. Utilize hash partitioning or range partitioning based on the specific characteristics of your data and the operations you plan to perform. Additionally, consider re-partitioning your data after certain transformations to ensure balanced workloads throughout your processing pipeline. Monitoring partition sizes and adjusting the number of partitions based on the data volume can also help maintain optimal performance.
Utilize In-Memory Computations
Leveraging Spark’s in-memory computing capabilities can dramatically reduce the latency of your data processing tasks. By caching datasets that are frequently accessed or used in iterative algorithms, you minimize the need for expensive disk I/O operations.
Use the cache()
method for datasets that fit entirely in memory and are used multiple times, or the persist()
method with different storage levels for more control over memory usage and fault tolerance. In-memory computations are particularly beneficial for machine learning algorithms and interactive data analysis, where speed is essential. Regularly review your caching strategy to ensure that the most beneficial datasets are cached, and uncache datasets that are no longer needed to free up memory.
Choose the Right Data Format
Selecting the appropriate data format for your Spark application can significantly impact both performance and storage efficiency.
Columnar storage formats like Parquet and ORC are optimized for read-heavy operations, offering efficient compression and encoding schemes that reduce storage space and improve I/O performance. These formats support column pruning and predicate pushdown, which can greatly accelerate query execution times by reading only the necessary data.
For write-heavy workloads, consider formats like Avro, which are designed for efficient data serialization and deserialization. Additionally, if your application involves complex data types or nested structures, these formats handle such scenarios more effectively than traditional row-based formats.
Optimize Transformations and Actions
Designing efficient transformations is key to ensuring optimal performance in Spark. Narrow transformations, such as map
, filter
, and mapPartitions
, operate on each partition independently and do not require data shuffling across the network, making them inherently more efficient.
Wide transformations like reduceByKey
, groupByKey
, and join
involve redistributing data across partitions, which can introduce significant overhead. To minimize this overhead, apply filters and aggregations as early as possible in your processing pipeline to reduce the volume of data that needs to be shuffled. Additionally, use combiners for associative operations to reduce the amount of data transferred during shuffles. Monitoring and tuning these transformations can lead to substantial performance gains.
Use Broadcast Variables and Accumulators
Broadcast variables and accumulators are tools in Spark that can optimize your application’s performance. Broadcast variables allow you to efficiently distribute a read-only copy of a large dataset to all worker nodes, preventing the need to send a copy with every task. This is particularly useful for operations that join a large RDD with a smaller dataset.
To use broadcast variables, simply call the broadcast
method on the dataset. Accumulators, on the other hand, provide a mechanism for aggregating information across tasks, such as counters or sums. They are particularly useful for implementing global counters or collecting debugging information. Use them judiciously to track metrics or gather aggregated results without introducing significant overhead.
Monitor and Tune Performance
Regular performance monitoring and tuning are essential for maintaining the efficiency of your Spark applications. The Spark UI provides valuable insights into job execution, including information on task durations, stage progress, and resource utilization. Use these metrics to identify bottlenecks, such as tasks that take significantly longer than others (stragglers) or excessive garbage collection times.
Adjust Spark configurations like executor memory, number of cores, and parallelism settings to optimize resource usage. Experiment with different configurations to find the optimal balance for your specific workload. Additionally, consider using external monitoring tools like Ganglia, Graphite, or custom dashboards to gain deeper insights into cluster performance and to automate alerting for potential issues.
Unlocking data insights: Apache Spark and Ocean for Apache Spark integration
Learn more about the power of integration with our product, Instaclustr. We bring the best of two worlds, Apache Spark and Ocean Protocol, together in a seamless and efficient data processing system. With Ocean for Apache Spark, developers can leverage the power of Apache Spark to perform complex data processing tasks on data from various sources within the Ocean Protocol network. This integration opens up new possibilities for data-driven applications, such as machine learning and analytics, by providing access to a wide range of diverse and valuable data sets.
Tap into a vast array of data sets, uncovering insights and possibilities that were previously out of reach:
- Harness the power of Apache Spark for complex data processing tasks
- Access and process data from various Ocean Protocol sources
- Maintain data privacy, security, and control
- Discover new insights and opportunities with big data analytics
Ready to explore the potential of your big data? For more information visit Ocean for Apache Spark.