What is Apache Spark?
Apache Spark is an open-source, distributed computing system for big data processing and analytics. It was developed to overcome the limitations of Hadoop’s MapReduce, such as inefficient data processing and latency issues. Spark’s in-memory computation and RDD (resilient distributed dataset) abstraction make it highly efficient and much faster compared to traditional Hadoop processing. Spark supports various high-level tools such as Spark SQL for structured data processing, MLlib for machine learning, and GraphX for graph processing.
Spark’s integration with Hadoop ecosystems and its flexibility make it a tool for a wide range of data processing tasks. Its capability to handle large datasets by distributing the computation tasks across multiple nodes has made it a preferred choice for many organizations.
How Apache Spark works
Apache Spark operates using a cluster computing model where tasks are distributed across multiple worker nodes. It leverages in-memory computation to optimize performance, storing intermediate data in memory, which significantly speeds up data processing tasks. Spark uses a master/worker architecture where the driver program that contains your main function launches parallel operations on a cluster of worker nodes.
The core of Spark lies in its abstraction of resilient distributed datasets (RDDs), which allow users to perform complex data manipulations. RDDs can be created from existing data in storage or transformed from other RDDs. Spark operations are divided into transformations and actions, optimizing execution through lazy evaluation. This means it doesn’t execute the transformations until an action is called, which helps to reduce redundancy and compute efficiencies in workflows.
Related content: Read our guide to architecture of Apache Spark (coming soon)
Tips from the expert
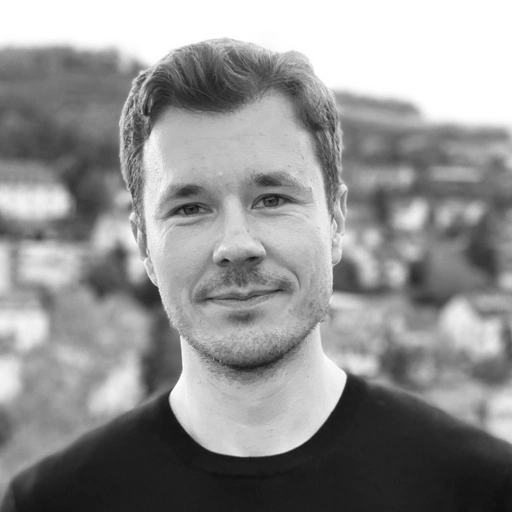
Merlin Walter
Solution Engineer
With over 10 years in the IT industry, Merlin Walter stands out as a strategic and empathetic leader, integrating open source data solutions with innovations and exhibiting an unwavering focus on AI's transformative potential.
In my experience, here are tips that can help you better utilize Apache Spark for your use cases:
- Optimize data partitioning: Partitioning is essential for parallelism, but you should also consider how your data will be read, joined, and queried to avoid data skew and reduce expensive shuffle operations. A well-partitioned dataset balances parallelism and partition size, ensuring efficient data processing. Avoid making users read through large volumes of data unnecessarily by planning partitions based on downstream usage and queries.
- Use Efficient Data Formats: I highly recommend using Apache Parquet as your go-to data format. Parquet’s columnar storage and excellent compression drastically improve performance, especially for analytical workloads. Its ability to support schema evolution also adds flexibility when working with changing data structures, and the built-in metadata allows Spark to optimize queries without scanning the entire dataset. Overall, I’ve found that Parquet provides the best balance of speed, efficiency, and scalability for Spark-based projects.
- Make use of broadcast variables: For read-only data that needs to be shared across multiple nodes, use broadcast variables. This reduces the overhead of data transfer and improves efficiency in tasks like joins with small lookup tables.
- Utilize accumulator variables: Accumulators are a way to perform a running total or count that can be safely updated across multiple nodes. Use them for counters or sums that need to be aggregated globally.
- Profile your jobs: Use Spark’s built-in profiling tools, like the Spark UI, to identify bottlenecks and optimize your Spark jobs. Tools like Ganglia or Graphite can also provide deeper insights into your cluster’s performance.
- Cache strategically: Use caching (
cache()
orpersist()
) for intermediate results that are reused multiple times. However, be mindful of memory usage and avoid over-caching, which can lead to memory spills and degrade performance.
Apache Spark use cases with code examples
1. Data Processing and ETL
Data processing and ETL (extract, transform, load) are critical components in data engineering workflows. Organizations need to extract data from various sources, transform it into a suitable format, and load it into a data warehouse or data lake for analysis.
How Spark can help:
Spark’s ability to handle large-scale data processing makes it ideal for ETL tasks. Its in-memory computation significantly speeds up the transformation processes, while its integration with various data sources simplifies data extraction and loading.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
from pyspark.sql import SparkSession spark = SparkSession.builder \ .appName('demo_app') \ .config("spark.hadoop.fs.s3.access.key", "XXXXXXXX") \ .config("spark.hadoop.fs.s3.secret.key", "XXXXXXXX") \ .config("spark.hadoop.com.amazonaws.services.s3.enableV4", "true") \ .config('spark.hadoop.fs.s3a.aws.credentials.provider', 'org.apache.hadoop.fs.s3a.SimpleAWSCredentialsProvider') \ .config("spark.hadoop.fs.s3.endpoint", "XXXXXXXXXXXXXXX") \ .config("spark.hadoop.fs.s3a.connection.establish.timeout","45000") \ .getOrCreate() # Extract data from a CSV file df = spark.read.csv("s3://bucket-name/data.csv", header=True, inferSchema=True) # Transform data by filtering and selecting relevant columns df_transformed = df.filter(df['age'] > 18).select("name", "age", "email") # Load data into a database df_transformed.write.format("jdbc").option("url", "jdbc:postgresql://<HOST>:<PORT>/<DB_NAME>?user=<DB_USER>&password=<DB_PASSWORD>"").option("dbtable", "adult_users").save() |
Here is a brief walkthrough of the code:
- A SparkSession is created, which is the entry point to using Spark.
- The data is extracted from a CSV file stored in an S3 bucket.
- The data is transformed by filtering out rows where the age is 18 or below and selecting specific columns (name, age, and email).
- The transformed data is loaded into a PostgreSQL database.
2. Stream Processing
Real-time data streams need to be processed on-the-fly for applications like live analytics, monitoring, and alerting systems.
How Spark can help:
Spark Streaming allows you to process live data streams, dividing the data into batches and processing them using Spark’s APIs.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
from pyspark.sql import SparkSession from pyspark.streaming import StreamingContext # Create a Spark session spark = SparkSession.builder \ .appName("SocketTextStream") \ .getOrCreate() # Create a StreamingContext with a batch interval of 10 seconds ssc = StreamingContext(spark.sparkContext, 10) # Create a DStream that will connect to hostname:port, e.g., localhost:9999 lines = ssc.socketTextStream("localhost", 9999) # Process the lines DStream by splitting the lines into words words = lines.flatMap(lambda line: line.split(" ")) # Map each word to a tuple (word, 1) pairs = words.map(lambda word: (word, 1)) # Reduce by key (word) to count the number of occurrences word_counts = pairs.reduceByKey(lambda x, y: x + y) # Print the resulting word counts word_counts.pprint() # Start the streaming computation ssc.start() # Wait for the streaming to finish ssc.awaitTermination() |
Here is a brief walkthrough of the code:
- A StreamingContext is created with a batch interval of 10 seconds.
- A DStream is created to read data from a TCP socket.
- Each line of data is split into words, and the words are counted.
- The word counts are printed in real-time.
3. Machine Learning and AI
Machine learning and AI applications often require processing large datasets to train and evaluate models.
How Spark can help:
Spark’s MLlib library provides scalable machine learning algorithms that can be easily integrated into your data pipeline. It supports various algorithms for classification, regression, clustering, and collaborative filtering.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
from pyspark.ml.classification import LogisticRegression from pyspark.ml.feature import VectorAssembler # Load data data = spark.read.csv("s3://bucket-name/data.csv", header=True, inferSchema=True) # Feature engineering assembler = VectorAssembler(inputCols=["feature1", "feature2"], outputCol="features") data = assembler.transform(data) # Train a logistic regression model lr = LogisticRegression(featuresCol='features', labelCol='label') model = lr.fit(data) # Predict predictions = model.transform(data) predictions.select("features", "label", "prediction").show() |
Here is a brief walkthrough of the code:
- Data is loaded from a CSV file.
- VectorAssembler is used to combine multiple feature columns into a single feature vector.
- A logistic regression model is trained using the prepared data.
- Predictions are made using the trained model, and the results are displayed.
4. Data Analytics
Enterprises need to analyze large datasets to derive business insights and make data-driven decisions.
How Spark can help:
Spark SQL provides an interface for structured data processing using SQL queries, making it easy to perform data analytics.
Example:
1 2 3 4 5 6 7 8 9 10 11 |
# Load data into a DataFrame df = spark.read.json("s3://bucket-name/data.json") # Register the DataFrame as a SQL temporary view df.createOrReplaceTempView("data_table") # Perform SQL query result = spark.sql("SELECT category, COUNT(*) as count FROM data_table GROUP BY category") # Show results result.show() |
Here is a brief walkthrough of the code:
- Data is loaded into a DataFrame from a JSON file.
- The DataFrame is registered as a temporary SQL view.
- A SQL query is executed to count the number of entries in each category.
- The results of the query are displayed.
5. Log Processing
Analyzing logs is essential for monitoring, troubleshooting, and improving system performance.
How Spark can help:
Spark’s ability to process large volumes of data quickly makes it suitable for log processing tasks.
Example:
1 2 3 4 5 6 7 8 9 10 11 |
# Load log data logs = spark.read.text("s3://bucket-name/logs/*.log") # Process logs to extract useful information error_logs = logs.filter(logs.value.contains("ERROR")) # Show the results error_counts = error_logs.count() print( f"Count Errors: {error_counts}") |
Here is a brief walkthrough of the code:
- Log data is loaded from multiple log files.
- Logs containing the keyword “ERROR” are filtered out.
- Errors are counted by their type.
- The results of the error counts are displayed.
6. Fog Computing
Fog computing involves processing data closer to where it is generated, reducing latency and bandwidth usage.
How Spark can help:
Spark’s architecture allows it to be deployed in a fog computing environment, processing data locally before sending aggregated results to a central server.
Example:
1 2 3 4 5 6 7 8 |
# Simulate local data processing on edge devices local_data = spark.read.json("file:///path/to/local/data.json") # Perform local aggregations local_aggregates = local_data.groupBy("sensor_id").agg({"value": "avg"}) # Send aggregated results to the central server local_aggregates.write.format("jdbc").option("url", "jdbc:mysql://central-server/db").option("dbtable", "aggregates").save() |
Here is a brief walkthrough of the code:
- Local data is loaded from a JSON file.
- Aggregations are performed on the local data (e.g., averaging sensor values).
- The aggregated results are sent to a central server for further processing.
7. Recommendation Systems
Recommendation systems are widely used in e-commerce and content platforms to provide personalized recommendations to users.
How Spark can help:
Spark’s MLlib includes collaborative filtering algorithms, such as ALS (alternating least squares), which can be used to build recommendation systems.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
from pyspark.ml.recommendation import ALS # Load user-item ratings data ratings = spark.read.csv("s3://bucket-name/ratings.csv", header=True, inferSchema=True) # Build the recommendation model using ALS als = ALS(userCol="userId", itemCol="movieId", ratingCol="rating") model = als.fit(ratings) # Generate top 10 movie recommendations for each user user_recommendations = model.recommendForAllUsers(10) # Show recommendations user_recommendations.show() |
Here is a brief walkthrough of the code:
- User-item ratings data is loaded from a CSV file.
- An ALS model is built using the loaded data.
- The model is used to generate the top 10 movie recommendations for each user.
- The recommendations are displayed.
8. Real-time Advertising
Real-time advertising requires processing user interactions in real-time to serve personalized ads.
How Spark can help:
Spark Streaming can process user interaction data in real-time, enabling dynamic ad placement based on user behavior.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
# Function to simulate getting ads based on user profiles def get_ad_for_user(user_profile): # Placeholder logic for selecting an ad based on the user profile return f"Ad_for_user_{user_profile['user_id']}" # Simulate real-time data stream for user interactions interactions = ssc.socketTextStream("localhost", 9999) # Process interactions to update user profiles user_profiles = interactions.map(lambda interaction: (interaction.user_id, interaction)) # Serve ads based on updated profiles ads = user_profiles.map(lambda profile: (profile[0], get_ad_for_user(profile[1]))) # Print the ads served ads.pprint() ssc.start() ssc.awaitTermination() |
Here is a brief walkthrough of the code:
- A real-time data stream for user interactions is simulated using a TCP socket.
- User profiles are updated based on interactions.
- Ads are dynamically served based on the updated profiles.
- The ads served are printed in real-time.
Unlocking the power of big data analytics with Ocean for Apache Spark
Ocean for Apache Spark is a powerful and versatile library that brings a range of benefits to developers working with Apache Spark. One of the key advantages of Ocean is its ability to seamlessly integrate with Spark, allowing users to leverage the full potential of Spark’s distributed computing capabilities while also benefiting from Ocean’s advanced features. By combining the strengths of both frameworks, Ocean enhances the data processing capabilities of Spark, enabling developers to tackle complex analytical tasks with ease.
One major benefit of Ocean for Apache Spark is its support for large-scale distributed deep learning. Ocean provides a high-level API that simplifies the process of training deep learning models on distributed Spark clusters. This allows developers to leverage the power of Spark’s distributed computing infrastructure to train models on massive datasets, significantly reducing the training time. Additionally, Ocean’s integration with popular deep learning frameworks like TensorFlow and PyTorch enables seamless integration of existing deep learning workflows with Spark, making it easier to incorporate deep learning into big data analytics pipelines.
Another advantage of Ocean for Apache Spark is its support for distributed data processing and analytics. Ocean extends Spark’s DataFrame API with additional functionality for distributed data manipulation and analysis. This includes support for distributed data structures like distributed arrays and distributed tensors, which enable efficient distributed computations on large-scale data. With Ocean, developers can perform complex distributed computations on large datasets without having to worry about data shuffling or memory constraints, resulting in improved performance and scalability.
Ready to take your Apache Spark operations to the next level? Explore support for Ocean for Apache Spark today and experience the simplicity and efficiency of our powerful framework.
For more information: